Blazor and Supabase, Authentication - Part 2
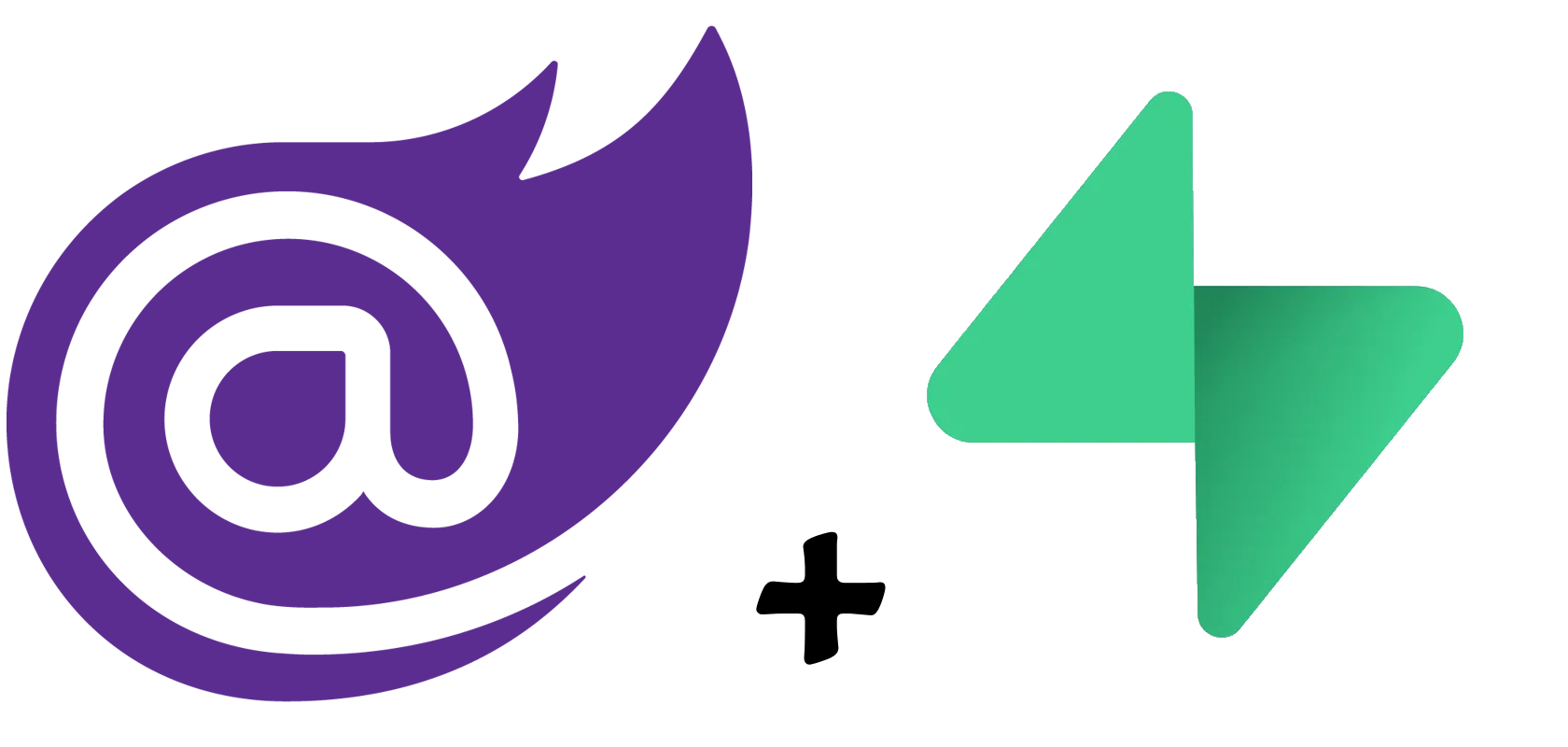
Part 1 : Database
Part 2 : Authentication
Part 3 : Real Time
Introduction:
One of the strengths of Supabase is its ability to handle all aspects of authentication and authorization for your application. Several providers are available, including email/password as well as most providers on the market (SAML, Microsoft, Facebook, Google, Apple, LinkedIn, ...).
Setting it up in a Blazor application wasn't very straightforward due to limited documentation on the topic. That's why I decided to create these articles. Some C# classes come from past personal projects or from classes I discovered on GitHub. But for you, it will be very easy to implement!
As before, don't forget to clone or browse the reference GitHub project: https://github.com/phnogues/Blazor.Supabase/
The authentication type I chose is using a JWT Token. There are many possibilities that I won’t describe here. Feel free to leave me a comment to learn more.
Let's get started!
Setup:
Here, I won’t copy the code, as it would be too hard to digest; take the time to browse the GitHub project.
Prerequisites:
Followed part 1 on setting up Supabase
Check that the information in your appsettings.json is correct.
- ValidIssuser : your Supabase API URL followed by /auth/v1
- ValidAudience : authenticated
- JwtSecret : your key, found in Project settings / API / JWT Secret
In Supabase, three pages will be important:
- The user database: Authentication
- Provider settings: Authentication / Providers
- Authentication settings: Project Settings / Authentication
In the code, I encourage you to look at the following classes:
- Program.cs
// Authenticated services
builder.Services.AddTransient<AuthService>();
builder.Services.AddTransient<UserService>();
builder.Services.AddScoped<AuthenticationStateProvider, SupabaseAuthenticationStateProvider>();
builder.Services.AddSingleton<IAuthorizationMiddlewareResultHandler, BlazorAuthorizationMiddlewareResultHandler>();
builder.Services.TryAddSingleton<IHttpContextAccessor, HttpContextAccessor>();
- SupabaseAuthenticationStateProvider: This will be our state manager for the JWT Token.
- AuthService: This class allows for logging in, registering, updating an account or password. The token will be persisted in local storage.
- In the RegisterAsync method, you can add as much additional data as you want, like first name or last name.
- For sending emails, I recommend using an SMTP (I use Brevo) since Supabase's free version has very limited email capabilities.
- UserService: Retrieves the logged-in user via their JWT Token.
- Routes.razor: Use a CascadingAuthenticationState tag.